The background of this cover image was published by Sara Torda on Pixabay, and I combined it with Vue icons to create the cover.
This article contains some thoughts and reflections I had while studying the relevant content of the Vue3 Tutorial | Runoob. The example code in the article comes from Runoob.
Vue sounds similar to "view," and its syntax is very close to the Jinja2 templating language in Flask. Vue determines the position through the element's id, creates an application using the createApp function, and mounts the application to the corresponding element with the mount function.
Hello, Vue!!#
For example, the following code uses mount(#app)
to mount HelloVueApp to the div element with id app, and the final result is displaying "Hello Vue!!" on the page.
<div id="app" class="demo">
{{ message }}
</div>
<script>
const HelloVueApp = {
data() {
return {
message: 'Hello Vue!!'
}
}
}
Vue.createApp(HelloVueApp).mount('#app')
</script>
Here, {{ }}
is used to output object properties and function return values, and {{ message }}
returns the message value in the application.
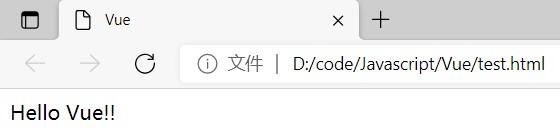
Hello Vue
Data Organization and Methods in Vue#
Data Option#
Data itself is a function and an essential part of the createApp function, returning an object. This object is wrapped by Vue through the reactive system and stored in the instance as $data
, where the data can be edited using methods similar to document.write
, as shown below:
const app = Vue.createApp({
data() {
return { count: 4 }
}
})
const vm = app.mount('#app')
document.write(vm.$data.count) // => 4
document.write("<br>")
document.write(vm.count) // => 4
document.write("<br>")
// Modifying vm.count will also update $data.count
vm.count = 5
document.write(vm.$data.count) // => 5
document.write("<br>")
// Conversely
vm.$data.count = 6
document.write(vm.count) // => 6
The result is shown in the following image:
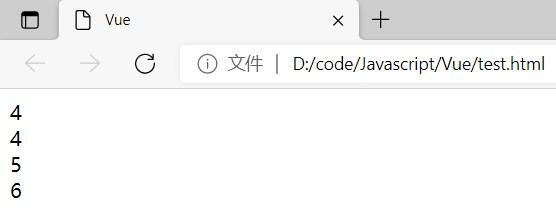
Data can be changed
Methods#
In Vue, methods can be added through the methods option, as shown below:
const app = Vue.createApp({
data() {
return { count: 4 }
},
methods: {
increment() {
// `this` refers to the component instance
this.count++
}
}
})
const vm = app.mount('#app')
document.write(vm.count) // => 4
document.write("<br>")
vm.increment()
document.write(vm.count) // => 5
It feels very close to object-oriented languages like Java and is easy to get started with, as shown in the result:
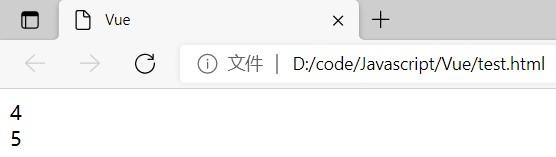
Using methods
Templates#
Text Interpolation#
As mentioned earlier, Vue is very similar to Jinja2, which is vividly reflected in templates. For example, in the following code:
<div id="app">
<p>{{ message }}</p>
</div>
In {{ message }}
, the message part will be converted to the specific value of this variable. When the value of the message variable changes in real-time, the information on the front end will also change in real-time. For example, here is a script code where the variable value changes after 10 seconds and reflects on the front end:
<script>
const HelloVueApp = Vue.createApp({
data() {
return {
message: 'Hello Vue!!'
}
}
})
const vm = HelloVueApp.mount('#hello-vue')
setTimeout("vm.message='lalala~'",10000)
</script>
Initially, the page displays Hello Vue!!
, and after 10 seconds, as the value of message changes, the displayed content changes to lalala~
, just like this gif:
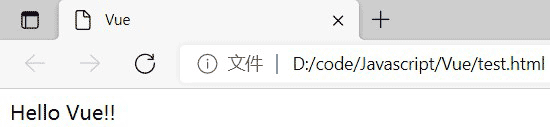
Variable value changes, front-end display changes
This is a form of one-way binding between data and the interface, where the display of interface elements changes with the variable value. One point to note here is that if you do not want the front-end element's content to change, you need to use the v-once
tag, such as <span v-once>{{ message }}</span>
.
Expressions#
In {{ }}
, JavaScript expressions can also be parsed, as shown in the following code:
<div id="app">
{{5+5}}<br>
{{ ok ? 'YES' : 'NO' }}<br>
{{ message.split('').reverse().join('') }}
<div v-bind:id="'list-' + id">Runoob Tutorial</div>
</div>
<script>
const app = {
data() {
return {
ok: true,
message: 'RUNOOB!!',
id: 1
}
}
}
Vue.createApp(app).mount('#app')
</script>
The displayed result is as follows:
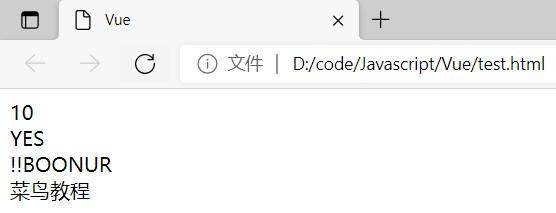
Processing of expressions
Directives#
Directives usually have a v-
prefix, and the v-once
mentioned above is one such directive. They are often used to reflect certain behaviors in the front-end interface when the value of a variable or expression changes. There are many types of directives, each achieving different functionalities, such as the following:
- Display Text (v-text)
Using the v-text
tag in elements like <p>
can display text corresponding to the variable value in data, as shown in the following example:
<div id='app'>
<p v-text="count"></p>
</div>
<script>
const app = Vue.createApp({
data() {
return { count: 4 }
},
})
const vm = app.mount('#app')
</script>
The displayed result is:
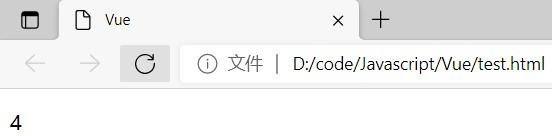
v-text directive
- HTML Interpolation (v-html)
Using the v-html
tag can also interpret and display HTML code. The following example shows the difference in displayed results between using text interpolation and using HTML output for the same line of code:
<div id="example1" class="demo">
<p>Using double curly braces for text interpolation: {{ rawHtml }}</p>
<p>Using v-html directive: <span v-html="rawHtml"></span></p>
</div>
<script>
const RenderHtmlApp = {
data() {
return {
rawHtml: '<span style="color: red">This will display in red!</span>'
}
}
}
Vue.createApp(RenderHtmlApp).mount('#example1')
</script>
The displayed result is:
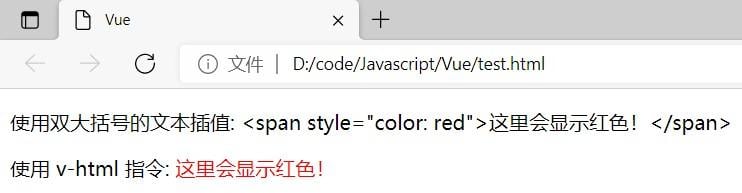
v-html directive
- Changing HTML Element's Attribute Values (v-bind)
For changing the values of attributes within HTML elements, Vue provides the v-bind
tag to handle attribute value changes. For example, the following code specifies class or id, where use is true, then class1 is used; otherwise, it is not used. To avoid involving other directives, I still use a delay to complete it.
<style>
.class1{
background: #66CCFF;
}
</style>
</head>
<body>
<div id="app">
<div v-bind:class="{'class1': use}">
v-bind:class directive
</div>
</div>
<script>
const app = {
data() {
return {
use: false
}
}
}
const vm = Vue.createApp(app).mount('#app')
setTimeout("vm.use=true",10000)
</script>
</body>
</html>
The actual effect:
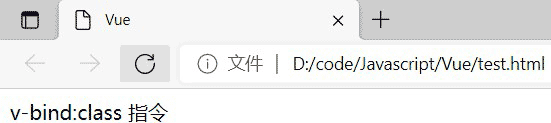
v-bind directive
You can see that after 10 seconds, the background color of the div changes because the value of use changes to true, making the class value become class1, thus applying the corresponding style of class1.
- Deciding Whether to Insert Elements (v-if)
For example, in the following example:
<div id="app">
<p v-if="seen">Now you see me</p>
</div>
<script>
const app = {
data() {
return {
seen: true /* Change to false, and the message will not be displayed */
}
}
}
Vue.createApp(app).mount('#app')
</script>
In this example, the v-if
directive will determine whether the element will be displayed based on the value of seen.
- Listening to DOM Events (v-on)
Used in conjunction with methods to handle front-end events, as shown in the following code:
<div id='app'>
<button v-on:click="welcome">Welcome</button>
</div>
<script>
const app = Vue.createApp({
data() {
return { count: 4 }
},
methods: {
welcome() {
alert("Hello")
}
}
})
const vm = app.mount('#app')
</script>
The actual effect:

v-on directive
If no parameters are passed, parentheses can be omitted.
- Two-Way Data Binding (v-model)
This directive is used to collect user input or selection for form controls (input, textarea, select, etc.), as shown in the following code:
<div id="app">
<p>{{ message }}</p>
<input v-model="message">
</div>
<script>
const app = {
data() {
return {
message: 'Runoob!'
}
}
}
Vue.createApp(app).mount('#app')
</script>
The significance of two-way binding is that user input on the page will modify the data in real-time, and the data in the data object will also be displayed in real-time on the user interface.
The actual effect:
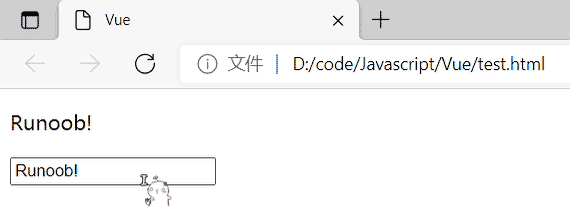
Processing of expressions
- Shortened Syntax for Two Directives
v-bind:
<!-- Full syntax -->
<a v-bind:href="url"></a>
<!-- Shortened -->
<a :href="url"></a>
v-on:
<!-- Full syntax -->
<a v-on:click="doSomething"></a>
<!-- Shortened -->
<a @click="doSomething"></a>